In 1970, the UNIX operating system developer Ken Thompson created the B language at Bell Labs. This B language later became the foundation for the programming language named BCPL (Basic Combined Programming Language), which was designed for a specific machine and system (DEC PDP-7 and UNIX). However, since it executed the code slowly, it was considered inadequate for developing an operating system.
In 1971, Denis Ritchie, from the Bell Labs team, produced a reliable, simple, easy-to-use C language that is compact and coherent. It further introduced some new concepts, such as the data types.
Later on, in early 1973, Denis Ritchie added a few more C features, like arrays, pointers and many more. The language was considered a portable high-level language. It became a procedural language. Procedural programs contain procedures, routines, subroutines, methods, or functions that contain a series of steps to perform a task. Any procedure could call any other procedure during the execution, including itself.
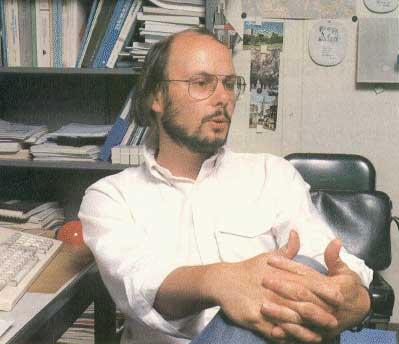
In early 1980, Bjarne Stroustrup, from Bell Labs, began developing “C with Classes”, also known as the C++ language. Stroustrup based C++ on C. He added object-oriented programming features and generic programming methodology to C language to develop C++. Therefore, C++ is considered the superset of C. Therefore, C++ software can also execute C code, and C++ can use all C libraries. The reasons why C++ has become so popular are as follows:
- The object-oriented features of C++ provide the ability to relate to the concepts involved in a problem.
- The features of C give the language the ability to relate to the hardware.
OBJECT-ORIENTED PROGRAMMING
The modular and structured approach to programming was followed before the development of object-oriented programming. However, a need for new methodology grew with the programs’ increasing size and complexity. This gave rise to the object-oriented programming methodology, which had the best-structured programming features with several new concepts. In this methodology, a program was organized around its code and data. Also, here, the data controlled the access to code. This approach reversed the conventional structured programming approach, where the code controlled the data.
In an object-oriented programming language like C++, the functions that act upon the data are defined. The major features of object-oriented programming are:
- Encapsulation
- Modularity
- Information Hiding and Abstraction
- Polymorphism
- Inheritance
Encapsulation
Encapsulation allows the binding of code and data and restricts any outside entity from misusing them. The bound code and data together form an object.
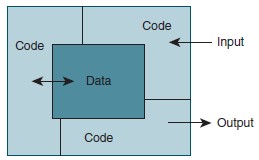
Hence, an object can be defined as a group of data variables and related methods/ routines. One particular object is called an instance. The code and data in an object may be public or private to that object. Private code or data is accessible only to the object it is placed, whereas any other part of the program may access public code or data.
This feature of object-oriented programming leads to two primary benefits in the development of programs:
- Modularity: The source code for an object can be independently maintained. Also, an object can be easily passed around in the system.
- Information hiding: The object can maintain private data and methods that can be changed at any time without affecting the other objects that depend on it. This feature of the C++ programming language is also known as abstraction.
Polymorphism
Poly means many, whereas morph means forms. Polymorphism in C++ programming language is the ability to use objects repeatedly without knowing their exact type at the compile time. A class is a collection of data members, and the member functions that operate on these data members. Since polymorphism refers to the same name granted to many forms, these forms may be classes or functions. As a result, polymorphism in C++ programming language is of two types:
- Class polymorphism
- Function polymorphism
Inheritance
Inheritance is a feature of C++ programming language in which one object can attain the properties of another object. A program can contain any number of classes. Similarly, multiple classes can be created in other programs. This might result in similar classes being defined across different programs to handle similar requirements. Therefore, to extend new classes from existing ones, C++ has the feature of inheritance, in which one class can be derived from another existing class, leading to the reuse of existing code. Inheritance is an essential feature of object-oriented programming.
C++ CHARACTER SET
C++ supports two types of character sets:
- The basic source character set
- The basic execution character set
The basic source character set in C++ has exactly 96 characters. They fit into 7 bits. These 96 characters include:
- All ASCII printing characters from 041 – 0177, except @ $ ` DEL
- Space
- Horizontal Tab
- Vertical Tab
- Form Feed
- Newline
These characters are used to compose a C++ source program.
The basic execution character set includes all the basic source character set characters and the control characters for alert, backspace, carriage return, and null. The execution character set is used when a C++ application is executing.
C++ TOKENS
A token is the smallest unit of a C++ program that has some meaning to the compiler. The C++ parser recognizes the following tokens:
Identifiers
An identifier is a sequence of characters (letters, numbers, and underscore) that we create to denote the following:
- Variable or an object name
- Class, structure, or union name
- Enumerated type name
- Members of a class, structure, union, or enumeration
- Function name
- typedef name
- Label name
- Macro name and macro parameter
The following characters are allowed for the first character of an identifier or any subsequent character:
_ | a | b | c | d | e | f | g | h | i | j | k | l | m |
N | o | p | q | r | s | t | u | v | w | x | y | z | |
A | B | C | D | E | F | G | H | I | J | K | L | M | |
N | O | P | Q | R | S | T | U | V | W | X | Y | Z |
Notice that the underscore (_) is considered as a letter here.
The following characters are allowed as any character in an identifier except the first:
0 | 1 | 2 | 3 | 4 | 5 | 6 | 7 | 8 | 9 |
Some limitations of C++ identifiers are as follows:
- The length of an identifier is not limited, although some compilers support only 32 characters.
- The identifiers created in C++ are case-sensitive. For example, the identifier name differs from the identifier Name or NAME.
- C++ identifiers should be declared before their usage.
- Identifiers cannot be re-declared in the same scope. However, the same name can be declared in other scopes.
- An identifier should always begin with a letter or an underscore.
- Spaces and marked letters are considered invalid in an identifier.
- Though identifiers can begin with an underscore (_), this should be avoided as the compiler may generate identifiers starting with an underscore for its purpose at implementation. This may give rise to an error.
- All identifiers that begin with an underscore and either an uppercase letter or another underscore are always reserved by C++.
- A keyword cannot be used as an identifier.
Following are some valid and invalid C++ identifiers:
int name; //valid
int NAME; //valid, but distinct from name
int nAmE; //valid, but distinct from name and NAME
int student_name; //valid
int _studentname; //valid, but reserved
int student-name; //invalid, can’t use a hyphen
int b4u; //valid
int 4u; //invalid, can’t start an identifier with a digit
int union; //invalid, can’t use a keyword
C++ Keywords
Keywords are the reserved identifiers that form the vocabulary of any programming language. They have special meanings and usage. The C++ keywords are case-sensitive and should be typed in lowercase only. The following table lists the C++ keywords:
asm | auto | bool | break |
case | catch | char | class |
const | const_cast | continue | default |
delete | do | double | dynamic_cast |
else | enum | explicit | Extern |
false | float | for | friend |
goto | if | inline | int |
long | mutable | namespace | new |
operator | private | protected | public |
register | reinterpret_cast | return | short |
signed | sizeof | static | static_cast |
struct | switch | template | this |
throw | true | try | typedef |
typeid | typename | union | unsigned |
using | virtual | void | volatile |
wchar_t | while |
We cannot use these keywords for purposes like creating a variable name.
Constants
Constants are those values that do not change over a period of time. In other words, they are fixed values or expressions. They are divided into:
- Integers
- floating-points
- characters and strings
C++ programming language supports two types of constants:
- Literal
- Symbolic
A literal constant is a value that is typed at any place in the program where it is needed. For example,
int height = 160;
A symbolic constant is a constant that has a name similar to that of the variable. Consider the following statement:
area_of_sq = 90 * 90;
In the above statement, 90 is a literal constant. This can be substituted with a symbolic constant in the following manner:
area_of_sq = side * side;
Integer constants identify integer decimal values, such as 12, 224, 999, etc. In addition to decimal numbers, C++ allows using octal numbers (base 8) and hexadecimal numbers (base 16) as literal constants. An octal number is preceded with a 0 (a zero character), and a hexadecimal number is preceded with dual characters 0x (zero, x) in such cases. For example, the following literal constants are all equivalent to each other:
90 // decimal 0132 // octal 0x5a // hexadecimal |
All the above represent the same number: 90 expressed as a decimal, octal and hexadecimal, respectively.
Literal constants have a specific data type. By default, integer literals are of type int. However, we can mark them as unsigned by appending the u character or long by appending l:
90 // int 90u // unsigned int 90l // long 90ul // unsigned long |
Floating point or real numbers denote numbers with decimals or exponents. They can include a decimal point, or an e character, or both a decimal point and an e character:
3.14159 // 3.14159 2.99 e+8 // 2.99 x 10^8 12 e-3 // 12 x 10^-3 |
Though the default type for floating-point literals is double, you can explicitly mark a float or a long double numerical literal:
2.999L // long double 2.99e8f // float |
Character constants can have a single character or a sequence of characters (strings). Whereas single character constants are enclosed between single quotes (‘), a string is enclosed between double quotes (“). If not written within these quotation marks, then those constants could be read as variables.
C++ allows representation of non-graphic characters, such as backspace, tab, carriage return etc., by using an escape sequence. An escape sequence represents a single character, always preceded by a backslash (\). The following table lists common escape sequences.
Escape Sequence | Non-graphic Character |
\0 | Null Character |
\a | Bell (beep) |
\f | Form feed |
\n | Newline |
\r | Carriage Return |
\t | Horizontal tab |
\v | Vertical tab |
Its numerical ASCII code can also express a character by preceding it with a backslash character (\). This ASCII code can be expressed as an octal (base-8) or hexadecimal (base-16) number. An example of an octal number representation is \26. In case of a hexadecimal representation, an x character is written before the digits: \x5A.
String literals are, by default, suffixed with the special character ‘\0′ (null), which denotes the string’s termination. Hence, the size of the string is always increased by one character. For example, the string “ALASKA” will be re-represented as “ALASKA\0” in the computer’s memory with the size of 7 characters.
Punctuators
The following characters are used as punctuators in C++. These are also called separators.
Asterisk * | Used as a multiplication operator or in pointer declaration. |
Braces { } | Opening and closing braces indicate the start and end of a compound statement. |
Brackets [ ] | Opening and closing brackets indicate array subscripts. |
Colon : | Opening and closing brackets indicate function calls, function parameters for grouping expressions, etc. |
Comma , | Used as a separator in a function argument list. |
Equal sign = | Used as an assignment operator. |
Parentheses ( ) | Opening and closing brackets indicate functions calls, function parameters for grouping expressions etc. |
Pound sign # | Used as pre-processor directive. |
Semicolon ; | Used as a statement terminator. |
Operators
Operators help in forming a defined link between the variables and constants. Operators in C++ are a set of keywords and signs. The number of operands required by the operator can classify operators. The operand is a value(s) or a variable(s) on which the computation is performed. For example,
5 + 4 has two operands (5 and 4) and one operator (+)
p + q – r has three operands (p, q and r) and two operators (+ and -)
-zz has one operand (zz) and one operator (-)
The operators that operate upon only one operand are referred to as unary operators, and the operators that operate between two operands are referred to as binary operators. For example,
5 + 4 is an example of a binary operator.
p + q – r is an example of a binary operator, where + is a binary operator for p and q, and –
is the operator for the value returned by (p + q) and r.
-zz is an example of the unary operator.
There are many types of operators defined in C++. Some common ones are as follows:
- Assignment operator ( = )
- Arithmetic operators (+, -, *, /, %)
- Compound assignment operators (+=, -=, *=, /=, %=)
- Relational operators ( ==, !=, >, <, >=, <= )
- Logical operators ( &&, || )
- Special operators
- Unary operators (*, &,!, ++, –, type, sizeof)
- Ternary operator ( ?: )
- Comma operator ( , )
- Scope operator (::)
- new and delete operators
Priority of operators
When an expression has several operands and operators, an order is established with each type of operator’s priority. From highest to lowest priority, the order is as follows:
Priority | Operator | Description | Associativity |
1 | :: | scope | Left |
2 | () [ ] -> . sizeof | Left | |
3 | ++ — | increment/decrement | Right |
! | unary NOT | ||
& * | Reference and Dereference (pointers) | ||
(type) | Typecasting | ||
+ – | Unary less sign | ||
4 | * / % | Arithmetical operations | Left |
5 | + – | Arithmetical operations | Left |
6 | < <= > >= | Relational operators | Left |
7 | == != | Relational operators | Left |
8 | && || | Logic operators | Left |
9 | ?: | Conditional | Right |
10 | = += -= *= /= %= | Assignation | Right |
11 | , | Comma, Separator | Left |